1. Python
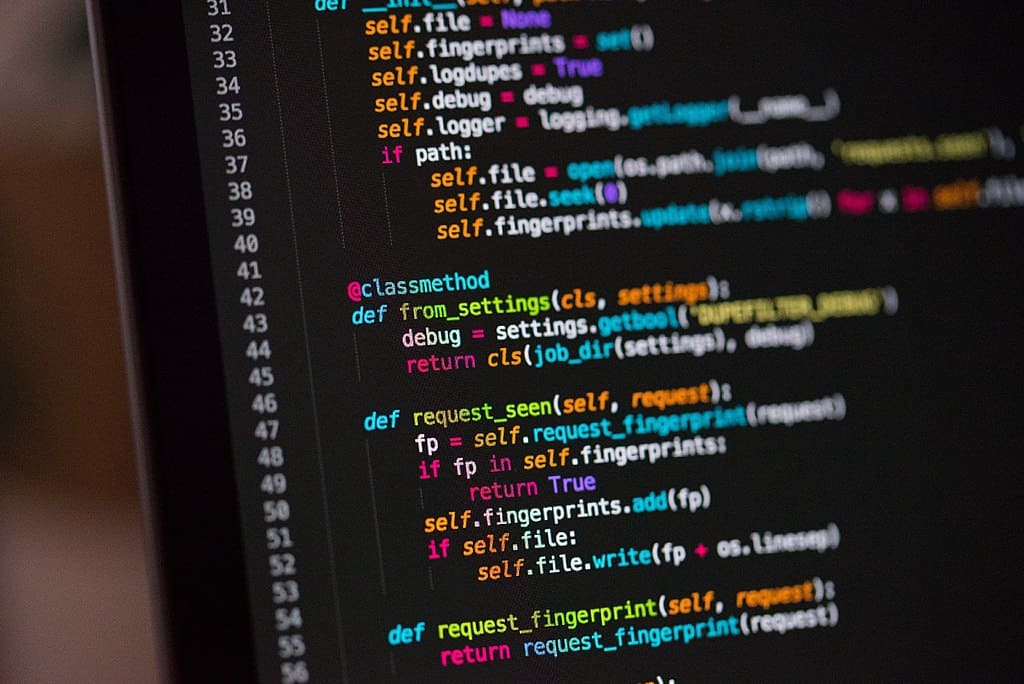
Python is an easy-to-learn programming language, with many documents and tutorials available.
1.1. Package Managers
1.2. Numpy
1.3. TensorFlow, Keras, and PyTorch
1.1. Package Managers
In Python, there are two major package managers, Pip and Anaconda, and we use both.
Here is the installation documents of them:
I primarily use Pip for package management.
1.2. Numpy
NumPy is a package for scientific computing in Python, and it is the de facto standard for doing numerical computing with matrices and vectors.
Tutorials:
1.3. Tensorflow, Keras, and PyTorch
There are three major frameworks in deep learning: TensorFlow, Keras, and PyThorch.
-
TensorFlow: An open-source deep learning framework. Since 2019’s release of TensorFlow 2, it has become more user-friendly. While TensorFlow 1 code remains supported, TensorFlow 2 is the recommended version.
-
Keras: A high-level API built on top of TensorFlow, and it shares a close relationship. Keras simplifies the implementation of common functionalities with less code, however, it may struggle with features that are not natively supported.
-
PyTorch: Like TensorFlow 2, PyTorch offers flexible implementation capabilities. It is a de facto standard in the academic field.
PyTorch was unstable on M1 Macs when I began development. Therefore, I chose to use Keras and TensorFlow in this document because my primary development machine is an M1 Mac.
However, I may move to PyTorch in the near future as exploring new academic technologies requires its use.
1.3.1. TensorFlow
If you use M1/M2 Mac, see the sites shown below:
1.3.2. Keras
Installing TensorFlow and its related modules is highly version-dependent.
Here’s how I installed it on M1 Mac.
[1] Installing Python 3.11.5 using pyenv
$ pyenv install 3.11.5
$ pyenv global 3.11.5
[2] Installing TensorFlow under venv
$ python3 -m venv ~/venv-metal
$ source ~/venv-metal/bin/activate
$ python -m pip install -U pip
$ pip install tensorflow==2.15.1
$ pip install tensorflow-metal==1.1.0
$ pip install matplotlib==3.9.0
1.3.3. Interconversion between Numpy and Tensorflow data
1.3.3.1. Numpy to Tensorflow
TensorFlow provides constant() method to convert from Numpy to TensorFlow.
>>> import numpy as np
>>> import tensorflow as tf
>>> N = np.arange(4).reshape(1,4)
>>> N
array([[0, 1, 2, 3]])
>>> N.shape
(1, 4)
>>> T = tf.constant(N)
>>> T
<tf.Tensor: shape=(1, 4), dtype=int64, numpy=array([[0, 1, 2, 3]])>
>>> type(T)
<class 'tensorflow.python.framework.ops.EagerTensor'>
1.3.3.2. Tensorflow to Numpy
TensorFlow also provides the numpy() method to convert from TensorFlow to NumPy.
>>> import numpy as np
>>> import tensorflow as tf
>>>
>>> t1 = tf.constant([5, 6, 7, 8])
>>> t1
<tf.Tensor: shape=(4,), dtype=int32, numpy=array([5, 6, 7, 8], dtype=int32)>
>>> n1 = t1.numpy()
>>> n1
array([5, 6, 7, 8], dtype=int32)
>>> type(n1)
<class 'numpy.ndarray'>
>>>
>>>
>>> t2 = tf.Variable([1, 2, 3, 4])
>>> t2
<tf.Variable 'Variable:0' shape=(4,) dtype=int32, numpy=array([1, 2, 3, 4], dtype=int32)>
>>> type(t2)
<class 'tensorflow.python.ops.resource_variable_ops.ResourceVariable'>
>>>
>>> n2 = t2.numpy()
>>> n2
array([1, 2, 3, 4], dtype=int32)
>>> type(n2)
<class 'numpy.ndarray'>
1.4. Scikit-learn
Scikit-learn is a tool set for predictive data analysis.